OpenGL continued with GLFW
OpenGL continued with GLFW
Hi Fellow Travellers,
Just some information about how I have things set up on my system.
We will now be playing with Visual C++ 2010 Express Beta2
http://www.microsoft.com/express/downloads/
Soon the official release will be available and we will be switching to it.
We will also be using a library called GLFW
http://www.glfw.org/
GLFW is a free, Open Source, multi-platform library for creating OpenGL contexts and managing input, including keyboard, mouse, joystick and time. It is intended to be simple to integrate into existing applications and does not lay claim to the main loop.
GLFW has native support for Windows, Mac OS X and many Unix-like systems using the X Window System, such as Linux and FreeBSD.
It is basically a better replacement for GLUT. (it actually works properly)
This package comes as a group of source files that have to be compiled.
The documentation has some faults in it, but as we plan to use GLFW linked into our code, rather than the DLL version of GLFW, we only have to compile the source and use the created "glfw.h", "glfw.lib" files. (use the release version of the "glfw.lib" file.
SETTING UP:
VisualC++ 2010 express when it installs will install all the require SDK files at
"C:\Program Files\Microsoft SDKs\Windows\v7.0A"
The search paths to these files will already be set up for you.
For convience we have coppied "glfw.h" into "C:\Program Files\Microsoft SDKs\Windows\v7.0A\Include\gl/" folder.
We have also coppied "glfw.lib" into "C:\Program Files\Microsoft SDKs\Windows\v7.0A\Lib\" folder.
Just some information about how I have things set up on my system.
We will now be playing with Visual C++ 2010 Express Beta2
http://www.microsoft.com/express/downloads/
Soon the official release will be available and we will be switching to it.
We will also be using a library called GLFW
http://www.glfw.org/
GLFW is a free, Open Source, multi-platform library for creating OpenGL contexts and managing input, including keyboard, mouse, joystick and time. It is intended to be simple to integrate into existing applications and does not lay claim to the main loop.
GLFW has native support for Windows, Mac OS X and many Unix-like systems using the X Window System, such as Linux and FreeBSD.
It is basically a better replacement for GLUT. (it actually works properly)
This package comes as a group of source files that have to be compiled.
The documentation has some faults in it, but as we plan to use GLFW linked into our code, rather than the DLL version of GLFW, we only have to compile the source and use the created "glfw.h", "glfw.lib" files. (use the release version of the "glfw.lib" file.
SETTING UP:
VisualC++ 2010 express when it installs will install all the require SDK files at
"C:\Program Files\Microsoft SDKs\Windows\v7.0A"
The search paths to these files will already be set up for you.
For convience we have coppied "glfw.h" into "C:\Program Files\Microsoft SDKs\Windows\v7.0A\Include\gl/" folder.
We have also coppied "glfw.lib" into "C:\Program Files\Microsoft SDKs\Windows\v7.0A\Lib\" folder.
Syddy........Just another breed of COOL CAT
Time to try a test program out and see if things are working properly.
Just start an empty project and create a new C++ file.
It will be noted that we are linking into the EXE file the following files ,"opengl32.lib", "glfw.lib", "user32.lib", without mucking around changing the properties for the project.
It is an easy way to do this as one builds up a project and has to add in more libraries as one progresses with the code.
If all is correct then a black filled window should be created when running the code.
One can also create a full screen mode and test it out by commenting/uncommenting the 2 appropriate lines.
In the next post we will look at how OpenGL looks at the 3D world and how simple objects can be placed into that world.
Just start an empty project and create a new C++ file.
Code: Select all
//must be in this order
#pragma comment(lib,"opengl32.lib")
#pragma comment(lib,"glfw.lib")
#pragma comment(lib,"user32.lib")
#include <GL/glfw.h>
int main( void )
{
int runningFlag = GL_TRUE;
glfwInit();// Initialize GLFW
if( !glfwOpenWindow( 800,600, 8,8,8,8,24,8, GLFW_WINDOW ) )// Open an OpenGL window
//if( !glfwOpenWindow( 800,600, 8,8,8,8,24,8, GLFW_FULLSCREEN ) )// Open OpenGL full screen
{
glfwTerminate();// Terminate if initialisation fails
return 0;
}
// Main loop
while( runningFlag == true )
{
// OpenGL rendering goes here...
glClear( GL_COLOR_BUFFER_BIT );
glfwSwapBuffers();
runningFlag = !glfwGetKey( GLFW_KEY_ESC ) && glfwGetWindowParam( GLFW_OPENED );// ESC key or Window Closed test
}
glfwTerminate(); // Close window and terminate GLFW
return 0;
}
It will be noted that we are linking into the EXE file the following files ,"opengl32.lib", "glfw.lib", "user32.lib", without mucking around changing the properties for the project.
It is an easy way to do this as one builds up a project and has to add in more libraries as one progresses with the code.
If all is correct then a black filled window should be created when running the code.
One can also create a full screen mode and test it out by commenting/uncommenting the 2 appropriate lines.
In the next post we will look at how OpenGL looks at the 3D world and how simple objects can be placed into that world.
Syddy........Just another breed of COOL CAT
We will now use an extra OpenGL Utility library that already exists in the SDK to make things a bit easier for us. "GlU32.lib"
Everytime we loop through the rendering loop it may be nice to clear the window with a particular chosen color.
This can be a default color and is set by the command
We also require a projection matrix. This is the matrix that defines the setup of the surface that we view with and render to.
Note that we have set it up to work on the projection matrix. We have loaded a Identity matrix that basically sets things up in a default positioned mode. we then set up the FRUSTUM for the view ... ie: we tell it what the vertical field of view of the window will be (65.0 deg), the windows aspect ratio (800.0 horizontal/600.0 vertical), the closest distance to the view point that things will be rendered from (1.0 units), the furthest distance from the view point that things will be rendered to (100.0 units).
here is the current setup code.
We should now be able to compile the code and get a sky blue window created.
Everytime we loop through the rendering loop it may be nice to clear the window with a particular chosen color.
This can be a default color and is set by the command
glClearColor( 0.0f, 0.5f, 0.9f, 0.0f ); // Preset a sky blue color for clear color
We also require a projection matrix. This is the matrix that defines the setup of the surface that we view with and render to.
Code: Select all
// Select and setup the projection matrix
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
gluPerspective( 65.0f, 800.0f/600.0f, 1.0f, 100.0f );
Note that we have set it up to work on the projection matrix. We have loaded a Identity matrix that basically sets things up in a default positioned mode. we then set up the FRUSTUM for the view ... ie: we tell it what the vertical field of view of the window will be (65.0 deg), the windows aspect ratio (800.0 horizontal/600.0 vertical), the closest distance to the view point that things will be rendered from (1.0 units), the furthest distance from the view point that things will be rendered to (100.0 units).
here is the current setup code.
Code: Select all
//must be in this order
#pragma comment(lib,"opengl32.lib")
#pragma comment(lib,"glfw.lib")
#pragma comment(lib,"user32.lib")
#pragma comment( lib, "GlU32.lib ") //will be now using GLU also
#include <GL/glfw.h>
int main( void )
{
int runningFlag = GL_TRUE;
glfwInit();// Initialize GLFW
if( !glfwOpenWindow( 800,600, 8,8,8,8,24,8, GLFW_WINDOW ) )// Open an OpenGL window
//if( !glfwOpenWindow( 800,600, 8,8,8,8,24,8, GLFW_FULLSCREEN ) )// Open OpenGL full screen
{
glfwTerminate();// Terminate if initialisation fails
return 0;
}
// Main loop
glClearColor( 0.0f, 0.5f, 0.9f, 0.0f ); // Preset a sky blue color for clear color
while( runningFlag == true )
{
// OpenGL rendering goes here...
glClear( GL_COLOR_BUFFER_BIT ); // Clear the window with the defined clear color
// Select and setup the projection matrix
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
gluPerspective( 65.0f, 800.0f/600.0f, 1.0f, 100.0f );
glfwSwapBuffers();
runningFlag = !glfwGetKey( GLFW_KEY_ESC ) && glfwGetWindowParam( GLFW_OPENED );// ESC key or Window Closed test
}
glfwTerminate(); // Close window and terminate GLFW
return 0;
We should now be able to compile the code and get a sky blue window created.
Syddy........Just another breed of COOL CAT
*** Important Information ***
When an OpenGL window is created with a default projection matrix then the following axis apply to the window created.
The middle of the screen is the 0,0,0 point.
Up direction is the positive Y axis.
Down direction is the negative Y axis.
Right direction is the positive X axis.
Left direction is the negative X axis.
Outward from the screen is the positive Z axis.
Into the screen is the negative Z axis.
These axis are different to what we have when DirectX is being used .... so be warned.
When an OpenGL window is created with a default projection matrix then the following axis apply to the window created.
The middle of the screen is the 0,0,0 point.
Up direction is the positive Y axis.
Down direction is the negative Y axis.
Right direction is the positive X axis.
Left direction is the negative X axis.
Outward from the screen is the positive Z axis.
Into the screen is the negative Z axis.
These axis are different to what we have when DirectX is being used .... so be warned.

Syddy........Just another breed of COOL CAT
Now is the time to try and display something simple to the viewport.
We will create a triangle at a Z axis location of -10 units.
REMEMBER we are looking along the -Z axis with the default viewport.....
The triangle will be created by passing the video card a color value and a vertex position for 3 vertecies making up a triangle.
bottom left vertex will be RED
bottom right vertex will be GREEN
top vertex will be BLUE
This is the code to do it
We go into model view mode.
Reset the starting point of the model to 0,0,0 and default rotation with the "glLoadIdentity();".
We then send the triangle information to the video card.
Here is the full code:
Note that we have created a slightly crooked bottom surfaced triangle with those vertices.
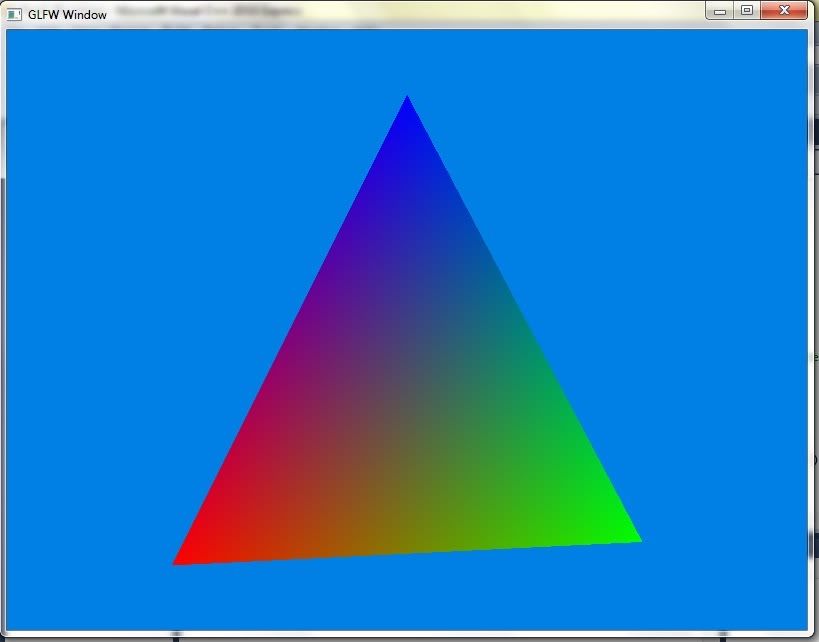
Notice how jaggie the bottom edge of the triangle happens to be.
We have not yet enabled A.A. for the screen.
Let us now try to turn on A.A. mode just before we actually create the window.
using "glfwOpenWindowHint( GLFW_FSAA_SAMPLES, 4);//ask for 4xAA"
Here is the full code:
Here is what the display now looks like **** note the smooth bottom edge of the triangle now.
EUREKA ..... AA works fine using ATI video cards .
We will create a triangle at a Z axis location of -10 units.
REMEMBER we are looking along the -Z axis with the default viewport.....
The triangle will be created by passing the video card a color value and a vertex position for 3 vertecies making up a triangle.
bottom left vertex will be RED
bottom right vertex will be GREEN
top vertex will be BLUE
This is the code to do it
Code: Select all
// Select and setup the modelview matrix
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
// Draw a colorful triangle
glBegin( GL_TRIANGLES );
//glBegin( GL_POLYGON ); // Could also use polygon instead
glColor3f( 1.0f, 0.0f, 0.0f );
glVertex3f( -5.0f, -5.0f, -10.0f ); //
glColor3f( 0.0f, 1.0f, 0.0f ); //
glVertex3f( 5.0f, -4.5f, -10.0f ); // Slight tilted triangle to create stepped bottom
glColor3f( 0.0f, 0.0f, 1.0f ); //
glVertex3f( 0.0f, 5.0f, -10.0f ); //
glEnd();
We go into model view mode.
Reset the starting point of the model to 0,0,0 and default rotation with the "glLoadIdentity();".
We then send the triangle information to the video card.
Code: Select all
// Draw a colorful triangle
glBegin( GL_TRIANGLES );
//glBegin( GL_POLYGON ); // Could also use polygon instead
glColor3f( 1.0f, 0.0f, 0.0f );
glVertex3f( -5.0f, -5.0f, -10.0f ); //
glColor3f( 0.0f, 1.0f, 0.0f ); //
glVertex3f( 5.0f, -4.5f, -10.0f ); // Slight tilted triangle to create stepped bottom
glColor3f( 0.0f, 0.0f, 1.0f ); //
glVertex3f( 0.0f, 5.0f, -10.0f ); //
glEnd();
Here is the full code:
Code: Select all
//must be in this order
#pragma comment(lib,"opengl32.lib")
#pragma comment(lib,"glfw.lib")
#pragma comment(lib,"user32.lib")
#pragma comment( lib, "GlU32.lib ") //will be now using GLU also
#include <GL/glfw.h>
int main( void )
{
int runningFlag = GL_TRUE;
glfwInit();// Initialize GLFW
if( !glfwOpenWindow( 800,600, 8,8,8,8,24,8, GLFW_WINDOW ) )// Open an OpenGL window
//if( !glfwOpenWindow( 800,600, 8,8,8,8,24,8, GLFW_FULLSCREEN ) )// Open OpenGL full screen
{
glfwTerminate();// Terminate if initialisation fails
return 0;
}
// Main loop
glClearColor( 0.0f, 0.5f, 0.9f, 0.0f ); // Preset a sky blue color for clear color
while( runningFlag == true )
{
// OpenGL rendering goes here...
glClear( GL_COLOR_BUFFER_BIT ); // Clear the window with the defined clear color
// Select and setup the projection matrix
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
gluPerspective( 65.0f, 800.0f/600.0f, 1.0f, 100.0f );
// Select and setup the modelview matrix
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
// Draw a colorful triangle
glBegin( GL_TRIANGLES );
//glBegin( GL_POLYGON ); // Could also use polygon instead
glColor3f( 1.0f, 0.0f, 0.0f );
glVertex3f( -5.0f, -5.0f, -10.0f ); //
glColor3f( 0.0f, 1.0f, 0.0f ); //
glVertex3f( 5.0f, -4.5f, -10.0f ); // Slight tilted triangle to create stepped bottom
glColor3f( 0.0f, 0.0f, 1.0f ); //
glVertex3f( 0.0f, 5.0f, -10.0f ); //
glEnd();
glfwSwapBuffers();
runningFlag = !glfwGetKey( GLFW_KEY_ESC ) && glfwGetWindowParam( GLFW_OPENED );// ESC key or Window Closed test
}
glfwTerminate(); // Close window and terminate GLFW
return 0;
}
Note that we have created a slightly crooked bottom surfaced triangle with those vertices.
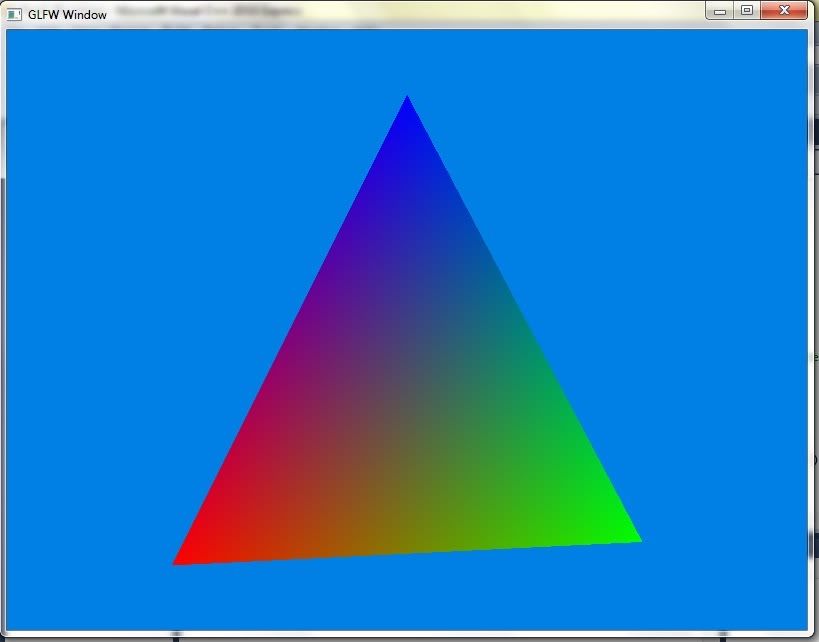
Notice how jaggie the bottom edge of the triangle happens to be.
We have not yet enabled A.A. for the screen.
Let us now try to turn on A.A. mode just before we actually create the window.
using "glfwOpenWindowHint( GLFW_FSAA_SAMPLES, 4);//ask for 4xAA"
Here is the full code:
Code: Select all
//must be in this order
#pragma comment(lib,"opengl32.lib")
#pragma comment(lib,"glfw.lib")
#pragma comment(lib,"user32.lib")
#pragma comment( lib, "GlU32.lib ") //will be now using GLU also
#include <GL/glfw.h>
int main( void )
{
int runningFlag = GL_TRUE;
glfwInit();// Initialize GLFW
glfwOpenWindowHint( GLFW_FSAA_SAMPLES, 4);//ask for 4xAA
if( !glfwOpenWindow( 800,600, 8,8,8,8,24,8, GLFW_WINDOW ) )// Open an OpenGL window
//if( !glfwOpenWindow( 800,600, 8,8,8,8,24,8, GLFW_FULLSCREEN ) )// Open OpenGL full screen
{
glfwTerminate();// Terminate if initialisation fails
return 0;
}
// Main loop
glClearColor( 0.0f, 0.5f, 0.9f, 0.0f ); // Preset a sky blue color for clear color
while( runningFlag == true )
{
// OpenGL rendering goes here...
glClear( GL_COLOR_BUFFER_BIT ); // Clear the window with the defined clear color
// Select and setup the projection matrix
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
gluPerspective( 65.0f, 800.0f/600.0f, 1.0f, 100.0f );
// Select and setup the modelview matrix
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
// Draw a colorful triangle
glBegin( GL_TRIANGLES );
//glBegin( GL_POLYGON ); // Could also use polygon instead
glColor3f( 1.0f, 0.0f, 0.0f );
glVertex3f( -5.0f, -5.0f, -10.0f ); //
glColor3f( 0.0f, 1.0f, 0.0f ); //
glVertex3f( 5.0f, -4.5f, -10.0f ); // Slight tilted triangle to create stepped bottom
glColor3f( 0.0f, 0.0f, 1.0f ); //
glVertex3f( 0.0f, 5.0f, -10.0f ); //
glEnd();
glfwSwapBuffers();
runningFlag = !glfwGetKey( GLFW_KEY_ESC ) && glfwGetWindowParam( GLFW_OPENED );// ESC key or Window Closed test
}
glfwTerminate(); // Close window and terminate GLFW
return 0;
}
Here is what the display now looks like **** note the smooth bottom edge of the triangle now.
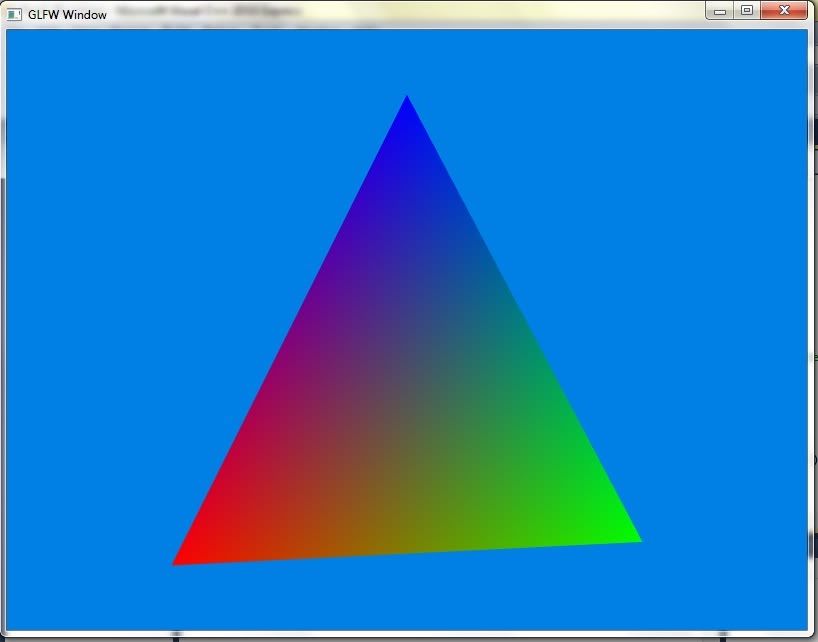
EUREKA ..... AA works fine using ATI video cards .

Syddy........Just another breed of COOL CAT
Have spent some time chasing up various Text rendering libraries for OpenGL, but before I start doing anything with them, I fealt it may be worthwhile to get some file distribution facility available so that I may be able to hoste some of this work for any people that may be interested.
I came across a file hosting site (free) at
http://www.mediafire.com
Opened up a free account so that files up to 200MB in size can be hosted free.
Should any one be (by chance) reading these posts, could they please see if they can download this file and run it.
http://www.mediafire.com/file/yjmzmkqdh ... Window.exe
Let us know if the download works correctly, and if the file actually runs.
Should things work properly, then I will host all the required library files with their matching header files so that others dont have to jump through all the various hoops to upgrade the created library files from VC++ ver6 up to the latest VC++ ver10.
I want to be able to host on a freely available hosting service rather than a limited access FTP server from the code group.
Syddy
I came across a file hosting site (free) at
http://www.mediafire.com
Opened up a free account so that files up to 200MB in size can be hosted free.
Should any one be (by chance) reading these posts, could they please see if they can download this file and run it.
http://www.mediafire.com/file/yjmzmkqdh ... Window.exe
Let us know if the download works correctly, and if the file actually runs.
Should things work properly, then I will host all the required library files with their matching header files so that others dont have to jump through all the various hoops to upgrade the created library files from VC++ ver6 up to the latest VC++ ver10.
I want to be able to host on a freely available hosting service rather than a limited access FTP server from the code group.
Syddy

Syddy........Just another breed of COOL CAT
Hi Nads,
Good to see you are still around.
Thanks for that information, I should have remembered that as I am using VisualC++ 2010, that people would also require the runtime DLL for that version of C. (not everyone is running the latest patched version of Win7)
Here it is.
http://www.mediafire.com/file/gkqhla4onjy/msvcr100.dll
Just pop it into the same location where that EXE is situated and things should work.
You could also drop that DLL file into "C:\Windows\System32" so that any program in future will be able to use it if you want.
Let us know if things work OK now.
Thanks
Good to see you are still around.
Thanks for that information, I should have remembered that as I am using VisualC++ 2010, that people would also require the runtime DLL for that version of C. (not everyone is running the latest patched version of Win7)
Here it is.
http://www.mediafire.com/file/gkqhla4onjy/msvcr100.dll
Just pop it into the same location where that EXE is situated and things should work.
You could also drop that DLL file into "C:\Windows\System32" so that any program in future will be able to use it if you want.
Let us know if things work OK now.

Thanks
Syddy........Just another breed of COOL CAT
Hi Syd, (apparently also known as Std......How did I relate that to you?
I did have a chuckle when I realized)
Appears to execute fine though on this machine takes about 8 secs to show on the screen the first time on this machine. P4 2.8Ghz Nvidia 7600gt
XTreme-G 197.13 XP 32bit drivers. 16XAA
Doesnt look as straight bottom edge as your picture above though, but
not too bad.
I did have a chuckle when I realized)
Appears to execute fine though on this machine takes about 8 secs to show on the screen the first time on this machine. P4 2.8Ghz Nvidia 7600gt
XTreme-G 197.13 XP 32bit drivers. 16XAA
Doesnt look as straight bottom edge as your picture above though, but
not too bad.
Hi Nads,
Thanks for that feedback.
The picture of the triangle bottom edge looks a little better than it will on an ordinary screen, because the *.JPG conversion of the screen capture has smeared a little smoother the bottom edge.
On the 12th April, within a few days time, the official release of VisualStudio 2010 will happen. Usually within a few days of that, they will also release VC++ 2010 Express ( the final release).
When that happens, I will create a new thread with links to all the files and setup information to put together a proper OpenGL game development environment with all the library files and configuration setup (similar to what was done for EAW).
This is why I required someone to confirm that the free file server, as well as the required download procedure was working properly.(thanks for your help).
Should you be interested in OpenGL programming then here is a great PDF file about it to look at.
http://www.mediafire.com/file/ytiztnkew ... 0Guide.pdf
Regards Syd
EDIT: Nads, are you still doing anything active with EAW development or are you just buggerising around like me?
Thanks for that feedback.
The picture of the triangle bottom edge looks a little better than it will on an ordinary screen, because the *.JPG conversion of the screen capture has smeared a little smoother the bottom edge.
On the 12th April, within a few days time, the official release of VisualStudio 2010 will happen. Usually within a few days of that, they will also release VC++ 2010 Express ( the final release).
When that happens, I will create a new thread with links to all the files and setup information to put together a proper OpenGL game development environment with all the library files and configuration setup (similar to what was done for EAW).
This is why I required someone to confirm that the free file server, as well as the required download procedure was working properly.(thanks for your help).
Should you be interested in OpenGL programming then here is a great PDF file about it to look at.
http://www.mediafire.com/file/ytiztnkew ... 0Guide.pdf
Regards Syd

EDIT: Nads, are you still doing anything active with EAW development or are you just buggerising around like me?
Syddy........Just another breed of COOL CAT
Hi Syd,
"EDIT: Nads, are you still doing anything active with EAW development or are you just buggerising around like me?"
Niether, just wafting about.
Downloaded the PDF you linked and got to this section in it.
I was nipped in the bud instantly
"EDIT: Nads, are you still doing anything active with EAW development or are you just buggerising around like me?"
Niether, just wafting about.
Downloaded the PDF you linked and got to this section in it.
I was nipped in the bud instantly

What You Should Know Before Reading This Guide
This guide assumes only that you know how to program in the C language and that you have some
background in mathematics (geometry, trigonometry, linear algebra, calculus, and differential
geometry). Even if you have little or no experience with computer−graphics technology, you should be
Here it is ....
Visual Studio 2010 express is now available for download.
http://www.microsoft.com/express/downloads/
This is the one that I would/will download (694MB in size so hopefully it will fit on a 700MB CD media)
http://www.microsoft.com/express/downloads/#2010-All
As it has all the languages on it including C++ and it is also an ISO file so a CD/DVD gets made from it. This allows for multiple installs or installs on multiple machines without repeated wasting of your internet download quota.
Now to see if my slow connection downloads it without stopping. (will take 6.5 hours on my 256k internet connection.
Time to get one of the spare machines loaded up with a clean version of windows, ready to install this and do some configuring and testing.

Visual Studio 2010 express is now available for download.
http://www.microsoft.com/express/downloads/
This is the one that I would/will download (694MB in size so hopefully it will fit on a 700MB CD media)
http://www.microsoft.com/express/downloads/#2010-All
As it has all the languages on it including C++ and it is also an ISO file so a CD/DVD gets made from it. This allows for multiple installs or installs on multiple machines without repeated wasting of your internet download quota.
Now to see if my slow connection downloads it without stopping. (will take 6.5 hours on my 256k internet connection.

Time to get one of the spare machines loaded up with a clean version of windows, ready to install this and do some configuring and testing.
Syddy........Just another breed of COOL CAT
Have you looked at SDL? The GLFW FAQ says "SDL is sometimes tricky to integrate into existing code and has never had OpenGL as its main focus", and I don't know how much existing code you have, but I don't think that not having OpenGL as its main focus is a problem. I have used SDL with OpenGL a bit and it was pretty easy - in 3 weeks of holidays, I went from knowing virtually nothing about SDL/OpenGL programming to having a tool that could partially display .3DX files from Microprose F-15 Strike Eagle III that ran fine on Linux and on Windows with MinGW.
Of course, once you're committed to one framework, I can't imagine you'd be too keen to change, but thought I'd mention SDL in case you aren't fully satisfied with GLFW! Conversely, I might take a look at GLFW one day
Regards,
David
Of course, once you're committed to one framework, I can't imagine you'd be too keen to change, but thought I'd mention SDL in case you aren't fully satisfied with GLFW! Conversely, I might take a look at GLFW one day

Regards,
David
Glad you found my little cubby hole where I plonk some of my random ramblings. 
Yes I did look at SDL, and in some of the early posts at stationX you may still find some posts where I was trying to integrate SDL into EAW to create new frontend code for the game. I could not get others to take any interest in working with SDL, and although I did make some small crappy frontend code samples ... just working by myself, I lost interest and went to other pursuits.
I liked SDL, but it appears that I much rather chase for knowledge, rather than actually push through and create something. This is why there are so many different disjointed topics in this little area.
Gosh, I have not posted much in a long time down here.

Yes I did look at SDL, and in some of the early posts at stationX you may still find some posts where I was trying to integrate SDL into EAW to create new frontend code for the game. I could not get others to take any interest in working with SDL, and although I did make some small crappy frontend code samples ... just working by myself, I lost interest and went to other pursuits.
I liked SDL, but it appears that I much rather chase for knowledge, rather than actually push through and create something. This is why there are so many different disjointed topics in this little area.
Gosh, I have not posted much in a long time down here.
Syddy........Just another breed of COOL CAT